Thursday, January 26, 2006
Nullable Value Types Irritation
I have to say working with these Nullable Value Types is a little irritating. Mainly because you can't go implcitly from int? to int and so although in the context of your Entity you may allow a null ID, the concept of a null ID may NOT be something you wish to expose to method calls using the object or at least avoid you having to type everything int? and then check hasValue.
Here's is what I am talking about.
You define a simple element entity as follows:
public class Element{
int? _id = null;
public int? ID
{
get { return _id;}
set {_id=value;}
}
}
You then may have a data object as follows:
public class MyData
{
public void Save(Element element)
{
if (element.ID == null)
SaveNew(...);
else
Update(...); }
}
The issue you now have is that any business methods, helper methods and so on that use this ID can then expect two types of query. The first is where you have a valid ID (number > 0) and the second is where you have a null value. Therefore, everytime you want to call a method such as the following, which will pass the ID of the element to the method you ALWAYS have to do hasValue.
public class MyBusiness
{
public void AttachToItem(int? id)
{
if (!id.hasValue)
throw new Exception("...");
//Do Something ....
}
}
Yes, before we had to do a check for -1 or some other magic number, but i'd rather there was an up front way of saying - in this certain scope it may be nullable, but outside it must have a value before it can be used as a parameter and the above method defined as follows:
public class MyBusiness
{
public void AttachToItem(int id) //i now have a valid int !
{
//Do Something ....
}
}
The only way i can think of solving this without writing loads of code, would be to have some kind of c#meta which allows you to put constraints on the variables in terms of scope, value, casting and exception.
So I may define my nullable int? as follows (making all this up now):
int? _id = null : valuecast(condition[value>0], IDException);
This basically says, define my _id variable as a nullable int? and when it is cast to an int value - [ in order to solving having to check for nulls in all my methods and so allow then to be defined as proper int value types ] - if the current value of _id is not greater than 0 then throw the user defined IDException.
Something like this would then provide me with a way of casting all of my fields for my entity throughout my entire application, which allows me to ensure that i do have a valid int (and it is NOT null) which is needed is almost all of my cases and my interfaces outside my entity can just use int's to define their interfaces.
Here's is what I am talking about.
You define a simple element entity as follows:
public class Element{
int? _id = null;
public int? ID
{
get { return _id;}
set {_id=value;}
}
}
You then may have a data object as follows:
public class MyData
{
public void Save(Element element)
{
if (element.ID == null)
SaveNew(...);
else
Update(...); }
}
The issue you now have is that any business methods, helper methods and so on that use this ID can then expect two types of query. The first is where you have a valid ID (number > 0) and the second is where you have a null value. Therefore, everytime you want to call a method such as the following, which will pass the ID of the element to the method you ALWAYS have to do hasValue.
public class MyBusiness
{
public void AttachToItem(int? id)
{
if (!id.hasValue)
throw new Exception("...");
//Do Something ....
}
}
Yes, before we had to do a check for -1 or some other magic number, but i'd rather there was an up front way of saying - in this certain scope it may be nullable, but outside it must have a value before it can be used as a parameter and the above method defined as follows:
public class MyBusiness
{
public void AttachToItem(int id) //i now have a valid int !
{
//Do Something ....
}
}
The only way i can think of solving this without writing loads of code, would be to have some kind of c#meta which allows you to put constraints on the variables in terms of scope, value, casting and exception.
So I may define my nullable int? as follows (making all this up now):
int? _id = null : valuecast(condition[value>0], IDException);
This basically says, define my _id variable as a nullable int? and when it is cast to an int value - [ in order to solving having to check for nulls in all my methods and so allow then to be defined as proper int value types ] - if the current value of _id is not greater than 0 then throw the user defined IDException.
Something like this would then provide me with a way of casting all of my fields for my entity throughout my entire application, which allows me to ensure that i do have a valid int (and it is NOT null) which is needed is almost all of my cases and my interfaces outside my entity can just use int's to define their interfaces.

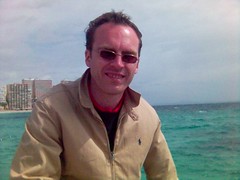






www.flickr.com |
Posts By Date
Profiles
- my taghop.org web
- taghop linkblogs
- todo list
- who i know
- flickr photos
- delicious links
- evdb events
- my things
- odeo subscriptions
NeighBloggers
taghop
Also made in Scotland
- Tarmac (John Loudon MacAdam)
- McIntosh Coat (Charles McIntosh)
- James Clerk Maxwell
- David Livingstone
- Television (John Logie Baird)
- BBC (Lord Reith)
- Kelvin Temperatures (William Thomson)
- RADAR (Sir Robert Alexander Watson-Watt)
- Steam Engine (James Watt)
- Americanism (John Witherspoon)
- Telephone (Alexander Graham Bell)
- Penicillin (Sir Alexander Fleming)
- Patron Saint of Ireland (Saint Patrick)
- Charles Rennie Mackintosh
- Celtic FC (Jock Stein)
- Treasure Island (Robert Louis Stevenson)
- Paddle Steamer (William Symington)
- Encylopaedia Britannica (William Smellie)
- Las Palmas Observatory (Charles Piazzi Smyth)
- The Bank of England (William Paterson)
- Logarithms & the Decimal Point (John Napier)
- The World Cup (Sir Thomas Lipton)
- MoreOver.com (David Galbraith)
- Blackboard (James Pillans)
- Liverpool FC (Bill Shankly)
- Iron Plough (James Small)
- Robinson Crusoe (Alexander Selkirk)
- Helium (Sir William Ramsay)
- Sociology (Adam Ferguson)
- Harry Potter
- Landspeed Record (Richard Noble)
- Hot Blast Oven (James Beaumont Neilson)
- Coal-Gas Lighting (William Murdock)
- Prime Minister of Canada (Sir John Alexander MacDonald)
- The Bicycle (Kirkpatrick Macmillan)
- Reflecting Telescope (James Gregory)
- The World's Worst Poet (William Topaz McGonagall)
- Geology (James Hutton)
- Carnegie Mellon (Andrew Carnegie)
- Carnegie Institution (Andrew Carnegie)
- Grandfather of the United States (Robert Dinwiddie)
- Universal Standard Time (Sir Sandford Fleming)
- Latent Heat & Carbon Dioxide (Joseph Black)
- James Bond (Sean Connery)
- Rob Stewart
- Auld Lang Syne (Robert Burns)
- Billy Connolly
- Annie Lennox
- U.S. Navy (John Paul Jones)
- Chariots of Fire (Eric Henry Liddell)
- Cure for Scurvy (James Lind)
- Tea Bags (Sir Thomas Lipton)
- Vacuum flask (Sir James Dewar)
- Postage Stamp (James Chalmers)
- Clerk Cycle Gas Engine (Sir Dugald Clerk)
- Cure for Malaria (George Cleghorn)
- Cure for Malaria (George Cleghorn)
- Groundskeeper Willie
- Peter Pan (Sir James Barrie)
- Kaleidoscope (Sir David Brewster)
- Toronto Globe (George Brown)
- Sherlock Holmes (Sir Arthur Conan Doyle)
- Graham's Law (Thomas Graham)
- The Wind in the Willows (Kenneth Grahame)
Release 2.0
Release 1.0
What I know
I Read
Archives
- July 17, 2005
- July 18, 2005
- July 19, 2005
- July 20, 2005
- July 21, 2005
- July 22, 2005
- July 27, 2005
- July 28, 2005
- August 01, 2005
- August 02, 2005
- August 03, 2005
- August 04, 2005
- August 07, 2005
- August 08, 2005
- August 09, 2005
- August 10, 2005
- August 11, 2005
- August 12, 2005
- August 13, 2005
- August 17, 2005
- August 19, 2005
- August 22, 2005
- August 24, 2005
- August 25, 2005
- August 27, 2005
- August 29, 2005
- August 30, 2005
- September 01, 2005
- September 02, 2005
- September 03, 2005
- September 04, 2005
- September 21, 2005
- September 22, 2005
- September 23, 2005
- September 30, 2005
- October 04, 2005
- October 06, 2005
- October 11, 2005
- October 14, 2005
- October 25, 2005
- October 27, 2005
- November 02, 2005
- November 08, 2005
- November 10, 2005
- November 12, 2005
- November 14, 2005
- November 16, 2005
- November 22, 2005
- December 02, 2005
- December 07, 2005
- December 23, 2005
- December 30, 2005
- January 02, 2006
- January 10, 2006
- January 11, 2006
- January 12, 2006
- January 14, 2006
- January 15, 2006
- January 16, 2006
- January 19, 2006
- January 20, 2006
- January 24, 2006
- January 25, 2006
- January 26, 2006
- January 30, 2006
- February 07, 2006
- February 08, 2006
- February 09, 2006
- February 20, 2006
- February 22, 2006
- February 23, 2006
- February 24, 2006
- February 27, 2006
- February 28, 2006
- March 01, 2006
- March 06, 2006
- March 08, 2006
- March 10, 2006
- March 13, 2006
- March 22, 2006
- March 24, 2006
- March 28, 2006
- March 29, 2006
- March 30, 2006
- March 31, 2006
- April 02, 2006
- April 06, 2006
- April 07, 2006
- April 13, 2006
- April 20, 2006
- April 26, 2006
- April 27, 2006
- April 28, 2006
- April 29, 2006
- April 30, 2006
- May 01, 2006
- May 02, 2006
- May 03, 2006
- May 04, 2006
- May 05, 2006
- May 07, 2006
- May 08, 2006
- May 10, 2006
- May 11, 2006
- May 15, 2006
- May 16, 2006
- June 02, 2006
- June 05, 2006
- June 06, 2006
- June 09, 2006
- June 11, 2006
- June 12, 2006
- June 13, 2006
- June 14, 2006
- June 20, 2006
- June 24, 2006
- June 26, 2006
- June 27, 2006
- June 29, 2006
- June 30, 2006
- July 01, 2006
- July 03, 2006
- July 08, 2006
- July 10, 2006
- July 12, 2006
- July 13, 2006
- July 25, 2006
- July 28, 2006
- August 01, 2006
- August 02, 2006
- August 05, 2006
- August 07, 2006
- August 08, 2006
- August 15, 2006
- August 22, 2006
- August 24, 2006
- August 27, 2006
- September 06, 2006
- September 07, 2006
- September 08, 2006
- September 11, 2006
- September 13, 2006
- September 14, 2006
- September 15, 2006
- September 21, 2006
- September 25, 2006
- October 02, 2006
- October 03, 2006
- October 25, 2006
- November 01, 2006
- November 10, 2006
- November 14, 2006
- November 15, 2006
- November 16, 2006
- November 17, 2006
- November 18, 2006
- November 20, 2006
- November 21, 2006
- November 29, 2006
- November 30, 2006
- December 08, 2006
- December 09, 2006